Intoducing while
Loop Statements
Now that you understand what a variable is, it is time to learn a powerful new kind of statement: a while
loop statement.
A while
statement, like an if
statement, is related to the control flow of a program. Control flow refers to where “control” or the next line of execution continues to next.
Like an if
statement, it has a boolean expression test condition and a body.
IMPORTANTLY, unlike an if
statement, when control finishes with the last line of the body, it jumps or loops back up to the boolean test expression again.
Try creating a new .py
file in your code editor with the following contents and playing with the argument value assigned to n
in the function call:
def print_0_to_n(n: int) -> int:
int = 0
counter: while counter < n:
print(counter)
= counter + 1
counter
print(f"Final: {counter}")
return counter
print(print_0_to_n(n=4))
Wow! That’s pretty neat, right? You have written a program which repeats what is inside the while
statement’s repeat block as many times as the n
argument provided. If you try producing a memory diagram of the above code listing, this is what you will find as the result:
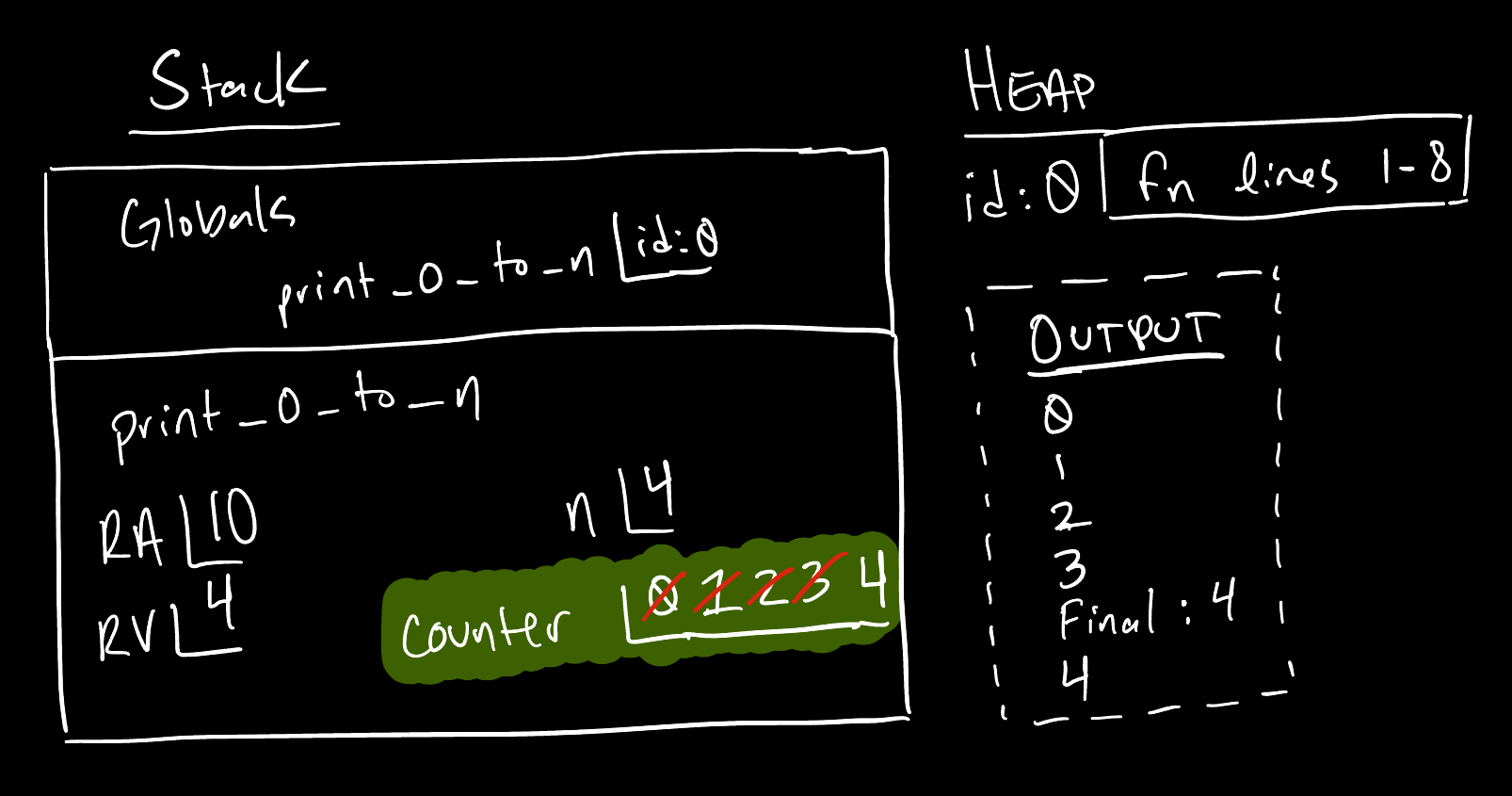
Notice with a while
loop, we are able to achieve repeating pattern. This is possible thanks to the combination of the counter
variable’s value being incremented by one each time the while
body reaches the line counter = counter + 1
, before jumping back to the test of counter < n
and repeating anew. Eventually, counter
’s value is incremented to no longer be less than n
and the loop completes. Control then jumps to the next line following the loop body, the print
statement, and finally returns the current value of counter
.
Iterating over a Sequence
Looping is more precisely called iteration when some value “iterates”, or makes small steps of progress, toward some goal. In the previous example, the counter
variable iterated toward n
.
Another use of iteration is iterating through the indices of a sequence. Recall a str
is a sequence of characters with ordered items addressed by a 0-based index using subscription notation: sequence[index]
.
Try adding another program in your code editor reverse.py
with the following contents. You are encouraged to type it out, rather than copy paste, to get a feeling for the syntax. The point of the program is to reverse a string by iterating through each of its characters and using concatenation in a clever way, by prefixing the result
string with each subsequent letter in the a_str
input string:
def reverse(a_str: str) -> str:
"""Reverse a string"""
int = 0
idx: str = ""
result: while idx < len(a_str):
= a_str[idx] + result
result = idx + 1
idx
return result
print(reverse(a_str="abc"))
You are once again encouraged to try producing a memory diagram of the above code listing. It will ultimately look like this:
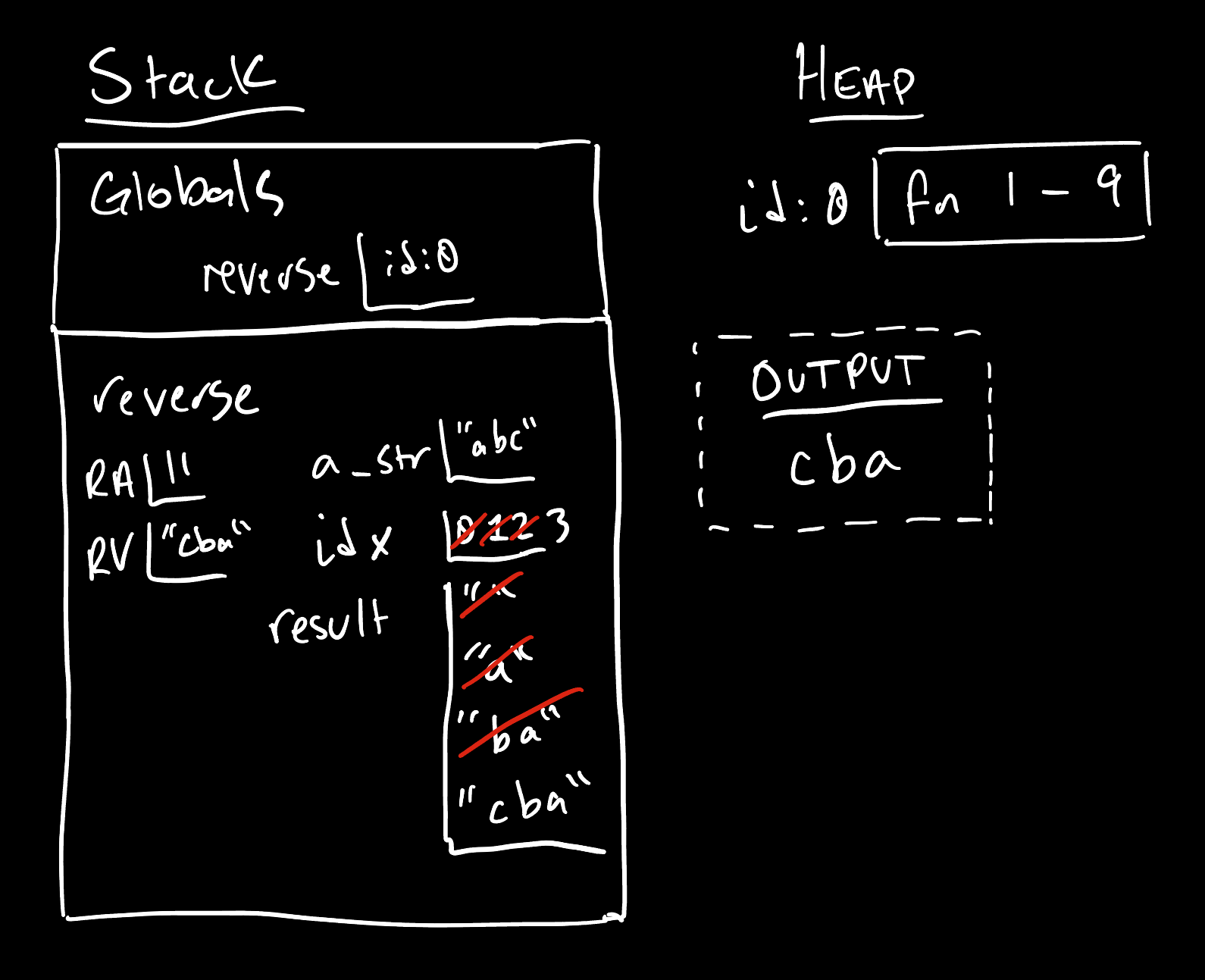
Notice how the idx
and result
variables are declared ahead of the while
loop and each serves a unique purpose. The idx
variable keeps track of the index at which the iterative process is working on. The result
variable keeps track of the “work in progress” result and updates it in the process of each iteration. Inside the loop body, importantly, the idx
variable is increasing and iterating its way toward being at least len(a_str)
, which is the loop’s exit condition.
We will continue exploring while
loops in more depth in the lessons and challenge questions ahead.